Numpy where function with two conditions
Numpy is a powerful library in Python used for numerical computations. One of the useful functions provided by Numpy is the where
function, which allows you to perform element-wise conditional operations on arrays. In this article, we will focus on how to use the where
function with two conditions.
When using the where
function with two conditions, both conditions must be boolean arrays of the same shape as the input array. The where
function will return elements from one of two input arrays based on the conditions provided.
Syntax of numpy.where()
numpy.where(condition1 & condition2, x, y)
Where:
condition1
andcondition2
are boolean arraysx
is the value to be returned when the condition is Truey
is the value to be returned when the condition is False
Now, let’s dive into some examples to understand how to use the Numpy where
function with two conditions:
Example 1
import numpy as np
arr = np.array([2, 5, 8, 10])
condition1 = (arr > 5)
condition2 = (arr < 10)
result = np.where(condition1 & condition2, arr, 0)
print(result)
Output:
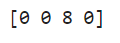
Example 2
import numpy as np
arr = np.array([[1, 3, 5], [7, 9, 11]])
condition1 = (arr % 2 == 0)
condition2 = (arr % 3 == 0)
result = np.where(condition1 & condition2, arr, -1)
print(result)
Output:
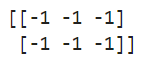
Example 3
import numpy as np
arr = np.array([[-1, 3, -5], [7, -9, 11]])
condition1 = (arr < 0)
condition2 = (arr % 2 != 0)
result = np.where(condition1 & condition2, arr, 100)
print(result)
Output:
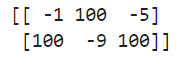
Example 4
import numpy as np
arr = np.array([[-1, 3, -5], [7, -9, 11]])
condition1 = (arr < 0)
condition2 = (arr > -10)
result = np.where(condition1 & condition2, arr, -arr)
print(result)
Output:
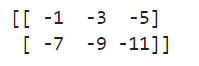
Example 5
import numpy as np
arr = np.array([[1, 3, 5], [7, 9, 11]])
condition1 = (arr % 2 == 0)
condition2 = (arr < 6)
result = np.where(condition1 & condition2, arr, arr * 2)
print(result)
Output:
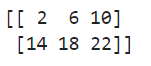
Example 6
import numpy as np
arr = np.array([[-1, 3, -5], [7, -9, 11]])
condition1 = (arr < 0)
condition2 = (arr > -5)
result = np.where(condition1 & condition2, arr * 5, arr + 10)
print(result)
Output:
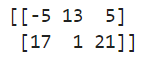
Example 7
import numpy as np
arr = np.array([[1, 3, 5], [7, 9, 11]])
condition1 = (arr % 3 == 0)
condition2 = (arr > 5)
result = np.where(condition1 & condition2, arr ** 2, arr)
print(result)
Output:
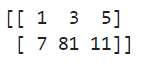
Example 8
import numpy as np
arr = np.array([[1, 3, 5], [7, 9, 11]])
condition1 = (arr % 2 == 0)
condition2 = (arr < 5)
result = np.where(condition1 & condition2, arr * 3, arr + 2)
print(result)
Output:
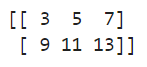
Example 9
import numpy as np
arr = np.array([[1, 3, 5], [7, 9, 11]])
condition1 = (arr % 2 != 0)
condition2 = (arr < 10)
result = np.where(condition1 & condition2, arr, arr // 2)
print(result)
Output:
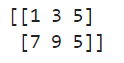
Example 10
import numpy as np
arr = np.array([[1, 3, 5], [7, 9, 11]])
condition1 = (arr % 3 == 0)
condition2 = (arr > 5)
result = np.where(condition1 & condition2, arr, arr + arr)
print(result)
Output:
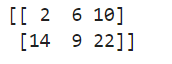
Conclusion of numpy.where()
These examples demonstrate how the Numpy where
function can be used with two conditions to perform element-wise operations on arrays. By applying multiple conditions, you can customize the output based on your specific requirements.